Getting Unstuck On Algorithms
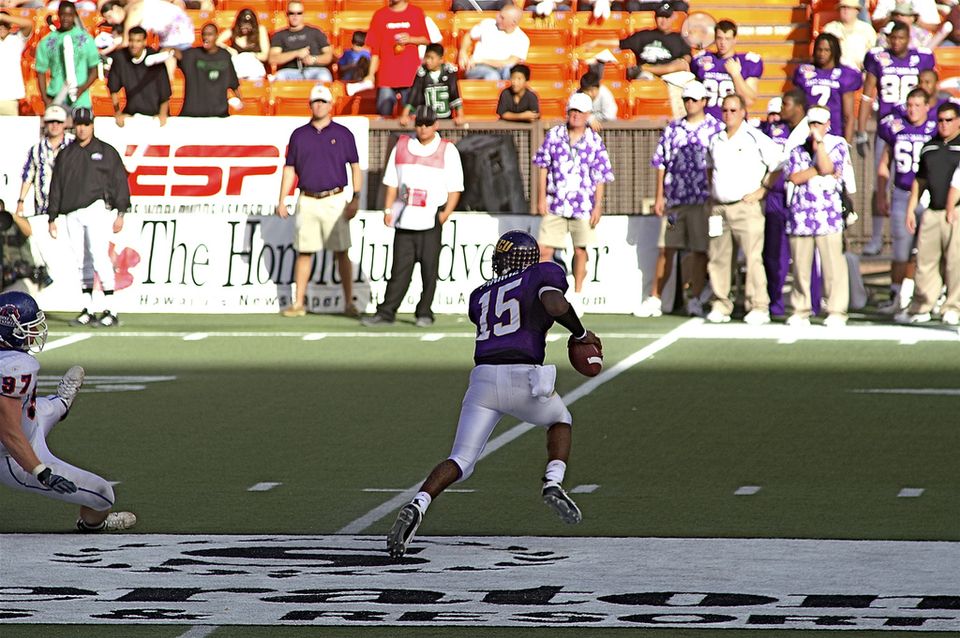
“Everyone has a plan: until they get punched in the face” – Mike Tyson
Interviews can be hard. To prep, ample time must be dedicated to studying different data structures, algorithms, and related questions. This serves you well until you inevitably get a question you have no idea how to solve. At that moment, you need a plan to get unstuck and solve the problem. Here is my algorithm to unstuck yourself.
First, take a deep breath. Your immediate goal is not the optimal solution. Your goal is to just make some progress. Like a quarterback whose pocket has collapsed - focus on the first down, not the touchdown. Often all you need is to make some progress to see a little bit further on the horizon - then the solution becomes clear.
TLDR;
- Clarify the question
- Brute-force
- Simplify the problem
- Spot inefficiencies
- Write out an example and walk through it
- Seek a base case
- Listen
Step 1: Clarify the question
Make sure you’re defining the problem and solving the question being asked. Before you freak out, understand exactly what is being asked. Some small tweaks in the question will take you down completely different paths.
Most problem-solving is happening at the subconscious level. Asking questions buys you some more time to really digest the problem and let your brain think.
Step 2: Brute-force
Now, you understand the problem but you still don’t see the optimal solution. Your best step here is to get an inefficient/brute-force solution. This will do a few things: 1. Give you confidence because you got something down 2. allow you to reason about the problem better 3. Give you a base for you and the interviewer to work from. Our brain is much better at thinking in iterations.
Note: sometimes it’s worth coding it out, other times not.
Step 3: Simplify the problem
Some of the examples you’re given may have edge cases and many input values to deal with. Let’s not reason about more data than we need. If there’s an array - just use a few values.
Also, let’s not focus on edge cases 'til we have a solution that makes sense. When we simplify, we can see the beginnings of a solution and that’s often all we need.
More often than not, solutions are <30 lines of code. KISS.
Step 4: Spot inefficiencies
Are you storing extra data? Are you repeating work? This line of questioning will allow you to focus on a simpler problem that’s easier to reason about when you’re stuck. You may find that the essence of the problem you’re facing rhymes with a problem you have solved in the past.
Look at where the inefficiency is. This is often a good time to think about 1-2 different data structures or common algorithms that are known tools for these types of problems. Is it an issue of time, space, or both? Can data be stored in a hashmap for easy lookup later? Is this work going to be redone and can be memoized? You get the deal.
This also might be a good time to go back to the question and reread it. Are there certain hints in the question? If the array of numbers is sorted, maybe it’s worth thinking about binary search, etc.
Step 5: Write out an example and walk through it
Write out a simplified example like mentioned above. Then step through iteration by iteration and talk through what is happening. This is great for the interviewer to see your thought process and will also help get your mind “running the program”. I’ve found this can often lead to a deeper understanding of the problem and my intuition can start helping out.
Step 6: Seek a base case
Many times problems are recursive. Take a step back and look at the problem - is this just the same problem being solved over and over again? If so, your brain will realize it may be a good case to use some recursive algorithm.
Step 7: Listen
Interviewers want you to succeed. If there is a roadblock they may give you some hints. When one is given, take a moment to think about the hint. Fully digest what was suggested, understand where they’re trying to take you, and why they would give that specific hint.
Summary:
To be a good engineer (and a good interviewee) you’re going to need to be a good problem solver. Many times you can’t or won’t solve problems off the bat, and that’s when you need to have a playbook. I encourage you to practice and seek out challenges that leave you in the land of the unknown. Take it one step at a time and you’ll often surprise yourself!
Note: You do need to have a great foundation in data structures and algorithms. I like Neetcode for dividing problems into topics. Problems become easier when you think in 2 steps: 1. Which kind of problem is this? 2. How do I use this solution to solve this specific problem?
Good luck and happy problem-solving!